Programming Ada: First Steps On The Desktop
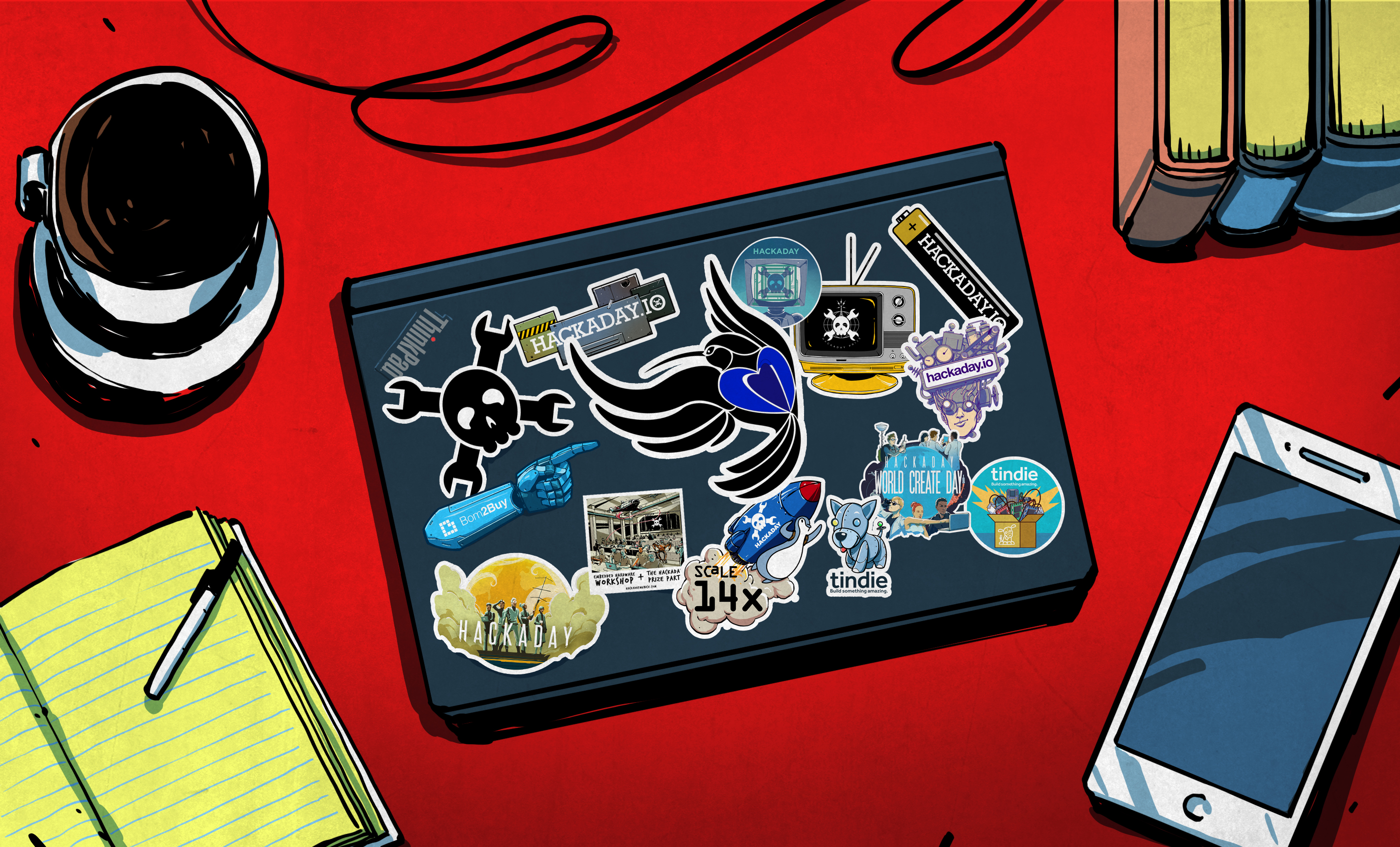
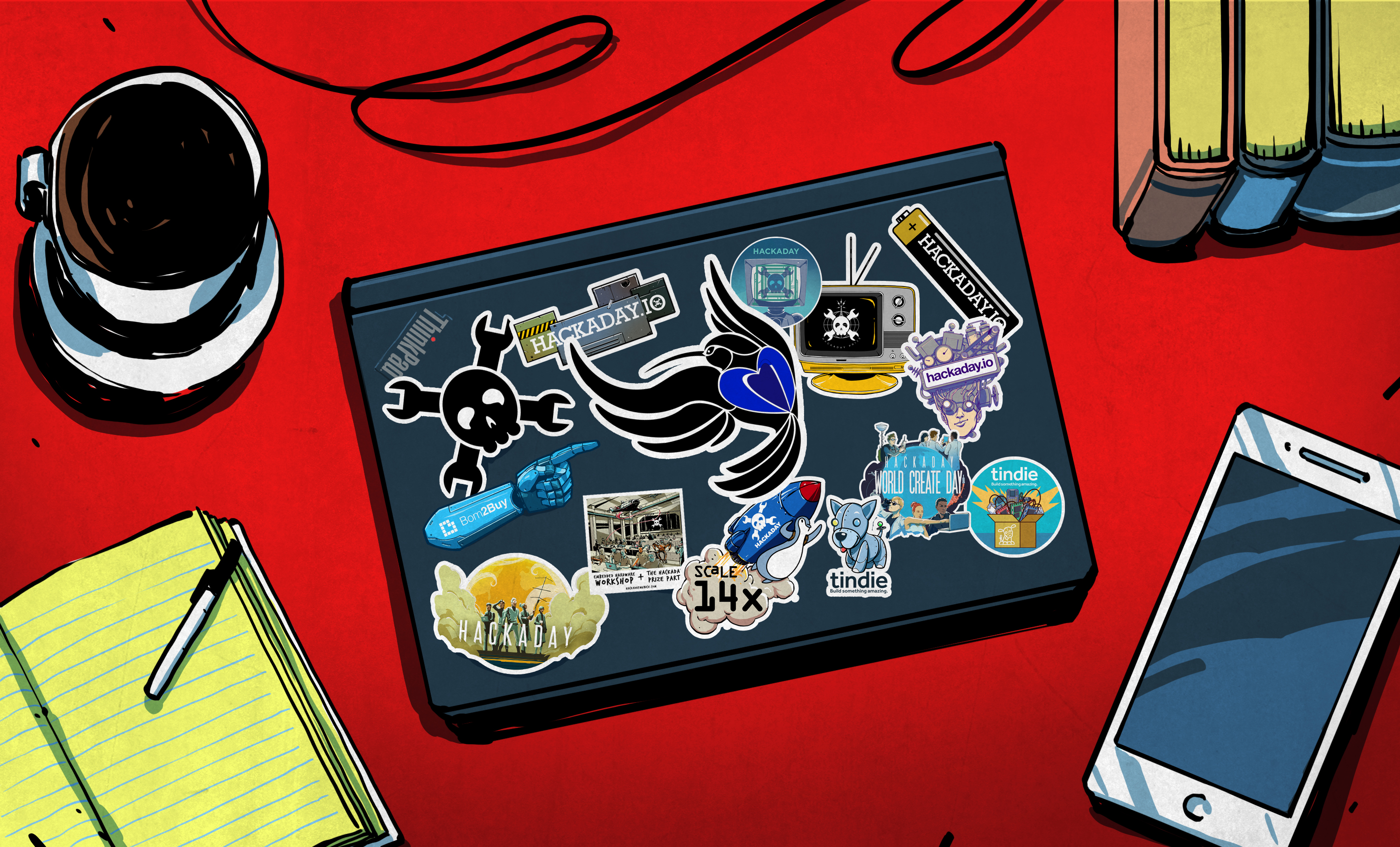
#Programming #Ada #Steps #Desktop
Who doesn’t want to use a programming language that is designed to be reliable, straightforward to learn and also happens to be certified for everything from avionics to rockets and ICBMs? Despite Ada’s strong roots and impressive legacy, it has the reputation among the average hobbyist of being ‘complicated’ and ‘obscure’, yet this couldn’t be further from the truth, as previously explained. In fact, anyone who has some or even no programming experience can learn Ada, as the very premise of Ada is that it removes complexity and ambiguity from programming.
In this first part of a series, we will be looking at getting up and running with a basic desktop development environment on Windows and Linux, and run through some Ada code that gets one familiarized with the syntax and basic principles of the Ada syntax. As for the used Ada version, we will be targeting Ada 2012, as the newer Ada 2022 standard was only just approved in 2023 and doesn’t change anything significant for our purposes.
Toolchain Things
The go-to Ada toolchain for those who aren’t into shelling out big amounts of money for proprietary, certified and very expensive Ada toolchains is GNAT, which at one point in time stood for the GNU NYU Ada Translator. This was the result of the United States Air Force awarding the New York University (NYU) a contract in 1992 for a free Ada compiler. The result of this was the GNAT toolchain, which per the stipulations in the contract would be licensed under the GNU GPL and its copyright assigned to the Free Software Foundation. The commercially supported (by AdaCore) version of GNAT is called GNAT Pro.
Obtaining a copy of GNAT is very easy if you’re on a common Linux distro, with the package gnat
for Debian-based distros and gcc-ada
if you’re Arch-based. For Windows you can either download the AdaCore GNAT Community Edition, or if you use MSYS2, you can use its package manager to install the mingw-w64-ucrt-x86_64-gcc-ada
package for e.g. the new ucrt64 environment. My personal preference on Windows is the MSYS2 method, as this also provides a Unix-style shell and tools, making cross-platform development that much easier. This is also the environment that will be assumed throughout the article.
Hello Ada
The most important part of any application is its entry point, as this determines where the execution starts. Most languages have some kind of fixed name for this, such as main
, but in Ada you are free to name the entry point whatever you want, e.g.:
with Ada.Text_IO; procedure Greet is begin -- Print "Hello, World!" to the screen Ada.Text_IO.Put_Line ("Hello, World!"); end Greet;
Here the entry point is the Greet
procedure, because it’s the only procedure or function in the code. The difference between a procedure and a function is that only the latter returns a value, while the former returns nothing (similar to void
in C and C++). Comments start with two dashes, and packages are imported using the with
statement. In this case we want the Ada.Text_IO
package, as it contains the standard output routines like Put_Line
. Note that since Ada is case-insensitive, we can type all of those names in lower-case as well.
Also noticeable might be the avoidance of any symbols where an English word can be used, such as the use of is
, begin
and end
rather than curly brackets. When closing a block with end
, this is post-fixed with the name of the function or procedure, or the control structure that’s being closed (e.g. an if/else block or loop). This will be expanded upon later in the series. Finally, much like in C and C++ lines end with a semicolon.
For a reference of the syntax and much more, AdaCore has an online reference as well as a number of freely downloadable books, which include a comparison with Java and C++. The Ada Language Reference Manual (LRM) is also freely available.
Compile And Run
To compile the simple sample code above, we need to get it into a source file, which we’ll call greet.adb
. The standard extensions with the GNAT toolchain are .adb
for the implementation (body) and .ads
for the specification (somewhat like a C++ header file). It’s good practice to use the same file name as the main package or entry point name (unit name) for the file name. It will work if not matched, but you will get a warning depending on the toolchain configuration.
Unlike in C and C++, Ada code isn’t just compiled and linked, but also has an intermediate binding step, because the toolchain fully determines the packages, dependencies, and other elements within the project before assembling the compiled code into a binary.
An important factor here is also that Ada does not work with a preprocessor, and specification files aren’t copied into the file which references them with a with
statement, but only takes note of the dependency during compilation. A nice benefit of this is that include
guards are not necessary, and headaches with linking such as link order of objects and libraries are virtually eliminated. This does however come at the cost of dealing with the binder.
Although GNAT comes with individual tools for each of these steps, the gnatmake
tool allows the developer to handle all of these steps in one go. Although some prefer to use the AdaCore-developed gprbuild
, we will not be using this as it adds complexity that is rarely helpful. To use gnatmake
to compile the example code, we use a Makefile which produces the following output:
mkdir -p bin mkdir -p obj gnatmake -o bin/hello_world greet.adb -D obj/ gcc -c -o objgreet.o greet.adb gnatbind -aOobj -x objgreet.ali gnatlink objgreet.ali -o bin/hello_world.exe
Although we just called gnatmake
, the compilation, binding and linking steps were all executed subsequently, resulting in our extremely sophisticated Hello World application.
For reference, the Makefile used with the example is the following:
GNATMAKE = gnatmake MAKEDIR = mkdir -p RM = rm -f BIN_OUTPUT := hello_world ADAFLAGS := -D obj/ SOURCES := greet.adb all: makedir build build: $(GNATMAKE) -o bin/$(BIN_OUTPUT) $(SOURCES) $(ADAFLAGS) makedir: $(MAKEDIR) bin $(MAKEDIR) obj clean: rm -rf obj/ rm -rf bin/ .PHONY: test src
Next Steps
Great, so now you have a working development environment for Ada with which you can build and run any code that you write. Naturally, the topic of code editors and IDEs is one can of flamewar that I won’t be cracking open here. As mentioned in my 2019 article, you can use AdaCore’s GNAT Programming Studio (GPS) for an integrated development environment experience, if that is your jam.
My own development environment is a loose constellation of Notepad++ on Windows, and Vim on Windows and elsewhere, with Bash and similar shells the environment for running the Ada toolchain in. If there is enough interest I’d be more than happy to take a look at other development environments as well in upcoming articles, so feel free to sound off in the comments.
For the next article I’ll be taking a more in-depth look at what it takes to write an Ada application that actually does something useful, using the preparatory steps of this article.